vtkRandomGraphSource can be used to create a random graph which includes points and edges.
vtkGraphLayoutView is a vtkRenderView which has its own renderer and iterator, we can use it to display graph view.
from vtk import * # create a random graph source = vtkRandomGraphSource() source.SetNumberOfVertices(100) source.SetNumberOfEdges(110) source.StartWithTreeOn() #create a view to display the graph view = vtkGraphLayoutView() view.SetLayoutStrategyToSimple2D() #SetLayoutStrategyToRandom() view.ColorVerticesOn() view.SetVertexColorArrayName("vertex id") view.SetRepresentationFromInputConnection(source.GetOutputPort()) # Apply a theme to the views theme = vtkViewTheme.CreateMellowTheme() view.ApplyViewTheme(theme) theme.FastDelete() #view.GetRenderWindow().SetSize(500,500) view.ResetCamera() view.Render() view.GetInteractor().Start()
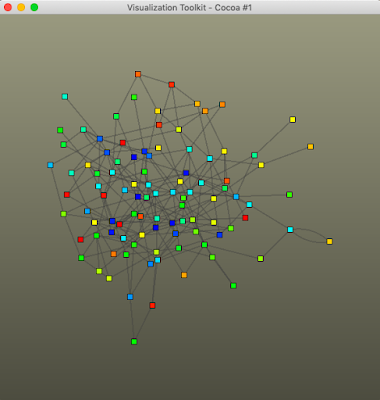
we can get the following result if the source starts with tree off:
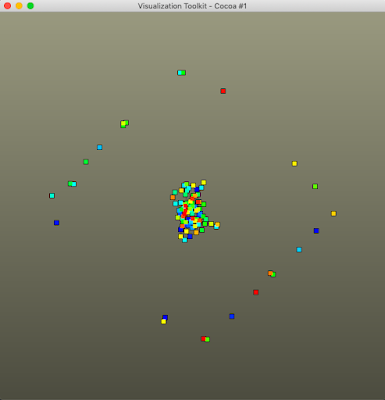
The vtkGraphLayoutView object can set different layout strategy.
There are some available layout strategies we can use: vtkFast2DLayoutStrategy, vtkCircularLayoutStrategy, vtkConeLayoutStrategy and etc.
vtkCircularLayoutStrategy example:
from vtk import * source = vtkRandomGraphSource() source.SetNumberOfVertices(100) source.SetNumberOfEdges(0) source.StartWithTreeOff() view = vtkGraphLayoutView() view.ColorVerticesOn() view.SetVertexColorArrayName("vertex id") view.SetRepresentationFromInputConnection(source.GetOutputPort()) strategy = vtkCircularLayoutStrategy() #Assigns points to the vertices around a circle with unit radius. view.SetLayoutStrategy( strategy ) theme = vtkViewTheme.CreateOceanTheme() view.ApplyViewTheme(theme) theme.FastDelete() view.ResetCamera() view.Render() view.GetInteractor().Start()
Others: