Rotate Vector To Orthogonal Direction
Just like in the picture, we will rotate vector to
which is orthogonal to the neighbor
. The vector
is the cross product of
and
.
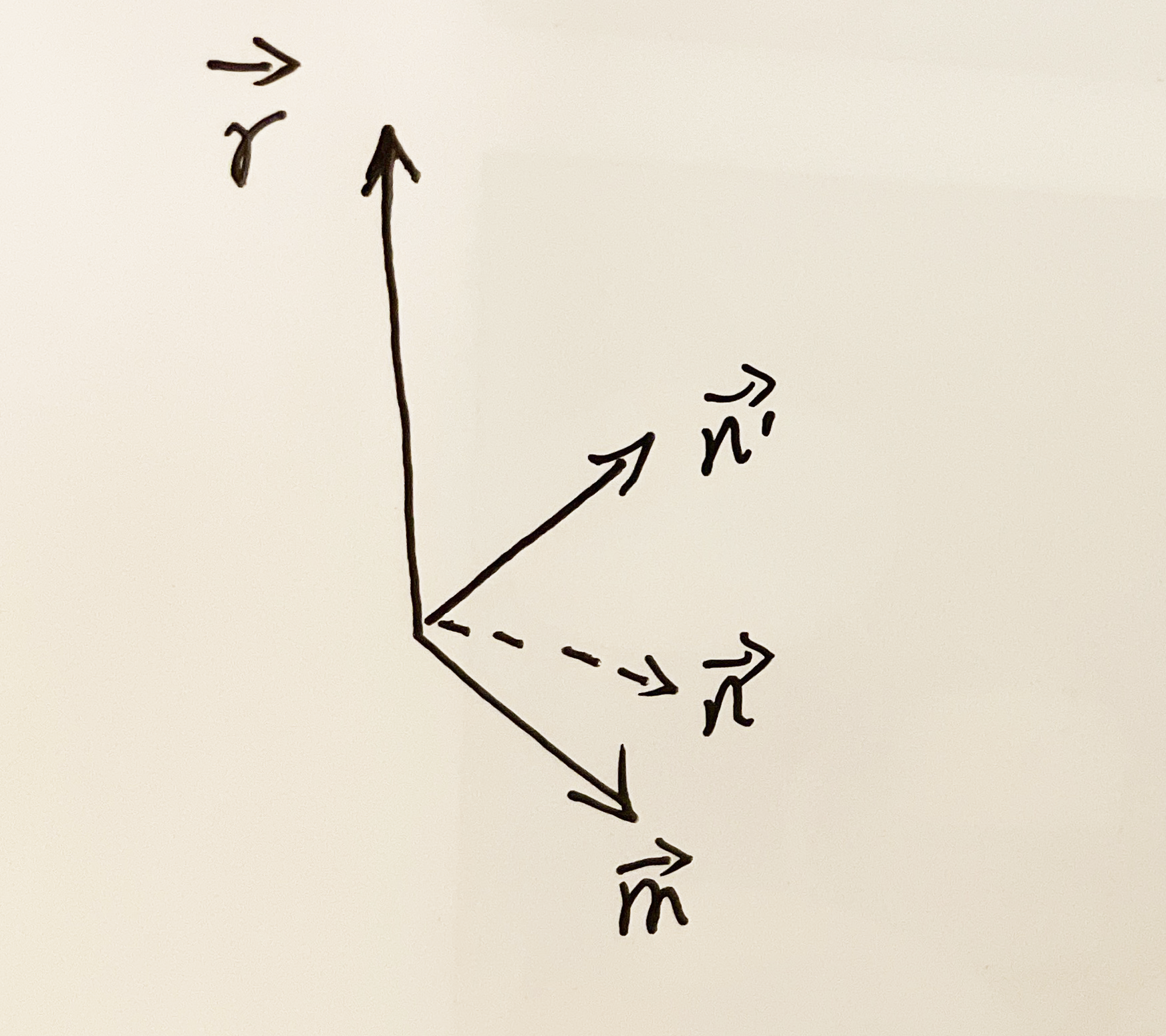
#include <iostream>
#include "point.hpp"
int main()
{
Point m( 1, 0, 0 );
Point n( 1, 0.5, 0 );
n.Unit();
Point r = m ^ n;
r.Unit();
n = r ^ m;
std::cout << "vec: " << n[0] << ", " << n[1] << ", " << n[2] << std::endl;
return 0;
}
C++ : Copy Subset Of Vector
std::vector<int> list1{ 1, 10, 2, 12, 34, 90, 11 };
std::vector<int> list2{ -1, -2, -3, -4 };
std::vector<int> tmp;
std::swap( list2, tmp );
std::copy( list1.begin() + 1, list1.begin() + 5, std::back_inserter(list2) );
for( auto value: list2 ){
cout << value << "\t";
}
Output:
10 2 12 34