The post shows how to use VTK to display 3D model with shading.
We need to make sure the version of VTK is equal to 9 or bigger than it.
To avoid the faceted appearance, compute points’ normal to support to has shading at triangle vertices.
Demo:
#include <iostream>
#include <vtkSmartPointer.h>
#include <vtkSphereSource.h>
#include <vtkActor.h>
#include <vtkConeSource.h>
#include <vtkSphereSource.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkPolyDataMapper.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkPolyDataNormals.h>
#include <vtkProperty.h>
#include <vtkCamera.h>
#include <vtkLight.h>
#include <vtkSTLReader.h>
#define vtkSPtr vtkSmartPointer
#define vtkSPtrNew(Var, Type) vtkSPtr<Type> Var = vtkSPtr<Type>::New();
using namespace std;
int main()
{
vtkSPtrNew( reader, vtkSTLReader );
reader->SetFileName( "string.stl" );
reader->Update();
vtkSPtrNew(polyDataNormals, vtkPolyDataNormals);
polyDataNormals->SetInputData( reader->GetOutput() );
polyDataNormals->ComputePointNormalsOn();
polyDataNormals->ComputeCellNormalsOff();
polyDataNormals->SetSplitting(false);
polyDataNormals->Update();
vtkSPtrNew( mapper, vtkPolyDataMapper );
mapper->SetInputConnection( polyDataNormals->GetOutputPort() );
vtkSPtrNew( actor, vtkActor );
actor->SetMapper( mapper );
#if (VTK_MAJOR_VERSION >= 9)
// Ambient 环境; diffuse 扩散; specular 镜面; coefficient 系数
actor->GetProperty()->SetInterpolationToPBR(); // shading interpolation: PBR
actor->GetProperty()->SetRoughness(0.1);
actor->GetProperty()->SetMetallic(0.05);
actor->GetProperty()->SetSpecular(0.5);
#endif
vtkSPtrNew( renderer, vtkRenderer );
renderer->AddActor(actor);
renderer->SetBackground( 0, 0, 0 );
vtkSPtrNew( renderWindow, vtkRenderWindow );
renderWindow->AddRenderer( renderer );
vtkSPtrNew( renderWindowInteractor, vtkRenderWindowInteractor );
renderWindowInteractor->SetRenderWindow( renderWindow );
renderer->ResetCamera();
renderWindow->Render();
renderWindowInteractor->Start();
return 0;
}
Output:
The faceted surface of model looks like:
vtkSPtrNew(polyDataNormals, vtkPolyDataNormals);
polyDataNormals->SetInputData( reader->GetOutput() );
polyDataNormals->ComputePointNormalsOff();
polyDataNormals->ComputeCellNormalsOn();
polyDataNormals->SetSplitting(false);
polyDataNormals->NonManifoldTraversalOff();
polyDataNormals->Update();
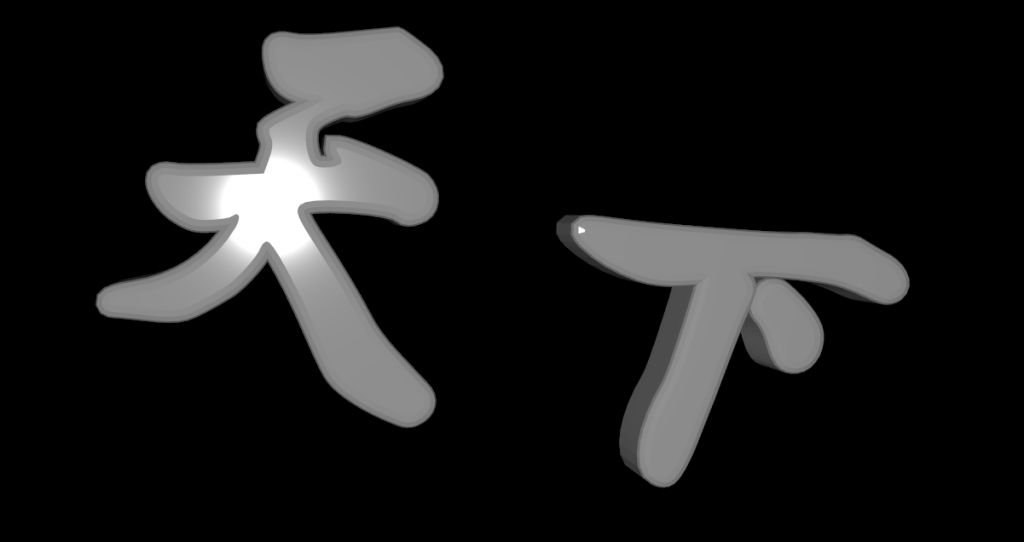