This article is a sequel to the previous one https://www.weiy.city/2023/04/draw-a-circle-around-mouse-postion-on-screen/.
Let’s check if the vertex of cone is inside the red circle when we move mouse.
CustomIteractorStyle.cpp
void CustomIteractorStyle::OnMouseMove()
{
//...
double pos[3] = { 0.5, 0, 0 };
m_Renderer->SetWorldPoint( pos[0], pos[1], pos[2], 0 );
m_Renderer->WorldToDisplay();
double *displayPos = m_Renderer->GetDisplayPoint();
m_Brush->CheckPtInsideCircle( displayPos[0], displayPos[1] );
}
UPaintBrush.cpp
#include "point.hpp"
vtkSmartPointer<vtkPoints2D> BrushItem::GetCirclePts()
{
vtkSmartPointer<vtkPoints2D> resultPts = vtkSmartPointer<vtkPoints2D>::New();
vtkNew<vtkTransform2D> tran;
tran->Translate(m_MousePos[0], m_MousePos[1]);
tran->TransformPoints(m_BrushPts, resultPts);
return resultPts;
}
UPaintBrush::UPaintBrush()
, m_CriclePts( nullptr )
void UPaintBrush::UpdateItem(double x, double y)
{
//...
m_CriclePts = brushItem->GetCirclePts();
}
void UPaintBrush::CheckPtInsideCircle(double x, double y)
{
Point pt( x, y, 0 );
bool sameDir = true;
for( int i = 0; i < m_CriclePts->GetNumberOfPoints()-2;++i )
{
Point pt0( m_CriclePts->GetPoint( i ) );
Point pt1( m_CriclePts->GetPoint( i+1 ) );
Point pt2( m_CriclePts->GetPoint( i+2 ) );
auto dir0 = (pt0 - pt)^(pt1 - pt);
auto dir1 = (pt1 - pt)^(pt2 - pt);
if( dir0.Dot( dir1 ) < 0 )
{
sameDir = false;
break;
}
}
Log( IInfo, "sameDir: ", sameDir );
}
UPaintBrush.h
public:
vtkSmartPointer<vtkPoints2D> GetCirclePts();
protected:
void CheckPtInsideCircle(double x, double y);
vtkSmartPointer<vtkPoints2D> m_CriclePts;
Output:
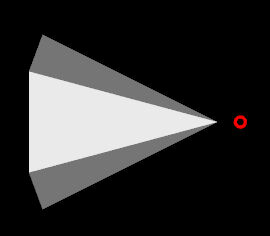
[Info][D:\code\vtk802\UPaintBrush.cpp:123]
sameDir: 0
[Info][D:\code\vtk802\UPaintBrush.cpp:123]
sameDir: 0
[Info][D:\code\vtk802\UPaintBrush.cpp:123]
sameDir: 0
[Info][D:\code\vtk802\UPaintBrush.cpp:123]
sameDir: 1
[Info][D:\code\vtk802\UPaintBrush.cpp:123]
sameDir: 1