Here are some folders which contain json files.
These json files have a version value, just look like the following image.
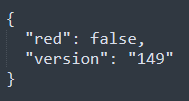
We will increase this number by 10 for every json file in current folders recursively.
Install command line tool jq
to modify json file easily.
Ubuntu: sudo apt-get install jq
Windows: just download exe on the page: https://github.com/jqlang/jq/releases
Jq Read
Command jq '.["foo"]'
Input {"foo": 42}
Output 42
Command jq '.foo'
Input {"foo": 42, "bar": "less interesting data"}
Output 42
Jq Remove Key
Command jq 'del(.foo)'
Input {"foo": 42, "bar": 9001, "baz": 42}
Output {"bar": 9001, "baz": 42}
Jq assignment
Command jq '.a = .b'
Input {"a": {"b": 10}, "b": 20}
Output {"a":20,"b":20}
Jq add key-value pair
cat fruits.json | jq '.fruit += {"source" : "India" }'
{
"fruit": {
"name": "apple",
"color": "green",
"price": 1.2,
"source": "India"
}
}
Reference page: https://jqlang.github.io/jq/manual/
I test my script on win10 in git-bash environment, you can replace jq-win64 with jq if you are on linux.
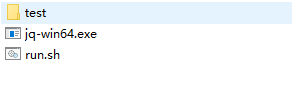
run.sh:
set -e
#folder=$1
folder="./"
files=()
echo "start to find json under folder: "$folder
find $folder -name "*.version.json" -print0 >tmpfile
while IFS= read -r -d $'\0'; do
files+=("$REPLY")
done < tmpfile
len=${#files[*]}
echo ${files[@]} #print all
echo "----- length is "${len}
echo ${files[1]}
#exit 0
# install jp on ubuntu firstly
for (( i=0; i<${len}; i++ ))
do
echo "input file: " ${files[$i]}
oldValue=`./jq-win64 -r '.["version"]' "${files[$i]}"`
echo $oldValue;
newValue=`expr $oldValue + 10`
echo $oldValue" "$newValue
./jq-win64 -r '.version = "'${newValue}'"' "${files[$i]}" > "${files[$i]}.new"
echo "${files[$i]}.new""===>""${files[$i]}"
mv "${files[$i]}.new" "${files[$i]}"
done