Here is a shell script which can help you to rename all files in the current folder.
You should input two strings as parameters for the script.
Usage example:
bash rename.sh ' ' '-'
It will rename all files which name contains space, these speaces will be changed to ‘-‘.
Rename.sh
#!/bin/bash
if [ $# -ne 2 ]; then
echo "Usage: $0 <old_string> <new_string>"
echo "Example: $0 'A4打印' 'printing on A4 paper'"
exit 1
fi
old_string="$1"
new_string="$2"
current_dir=$(pwd)
for file in "$current_dir"/*; do
if [ -f "$file" ]; then
filename=$(basename "$file")
new_filename=$(echo "$filename" | sed "s/$old_string/$new_string/g") # $old_string can be regex_pattern
if [ "$filename" != "$new_filename" ]; then
mv "$file" "$current_dir/$new_filename"
echo "Renamed: $filename -> $new_filename"
fi
fi
done
echo "Batch renaming completed."
You can also use regular expression for the old string parameter!
Enjoy it:
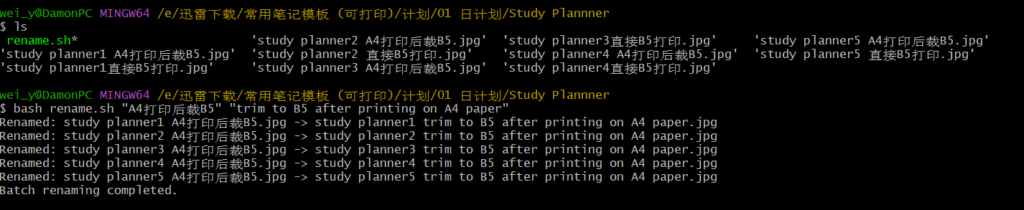