The post shows how to display bounding box of 3D model by lines and surfaces.
vtkSPtr<vtkPolyData> GetBoundsFacePolyData(double bounds[6])
{
double x[8][3]={{bounds[0],bounds[2],bounds[4]}, {bounds[1],bounds[2],bounds[4]}, {bounds[1],bounds[3],bounds[4]}, {bounds[0],bounds[3],bounds[4]},
{bounds[0],bounds[2],bounds[5]}, {bounds[1],bounds[2],bounds[5]}, {bounds[1],bounds[3],bounds[5]}, {bounds[0],bounds[3],bounds[5]}};
vtkIdType pts[6][4]={{0,1,2,3}, {4,5,6,7}, {0,1,5,4},
{1,2,6,5}, {2,3,7,6}, {3,0,4,7}};
vtkSPtrNew( points, vtkPoints );
vtkSPtrNew( polys, vtkCellArray );
vtkSPtrNew( polyData, vtkPolyData );
for (int i=0; i<8; i++) points->InsertPoint(i,x[i]);
for (int i=0; i<6; i++) polys->InsertNextCell(4,pts[i]);
polyData->SetPoints(points);
polyData->SetPolys(polys);
return polyData;
}
vtkSPtr<vtkPolyData> GetBoundsPolyData(double bounds[6])
{
std::vector<Point> pts;
for( int i = 0; i < 2; ++i )
{
for( int j = 2; j < 4; ++j )
{
for( int k = 4; k < 6; ++k )
{
pts.push_back( Point( bounds[i], bounds[j], bounds[k] ) );
}
}
}
vtkSmartPointer<vtkPolyData> boundsPolydata =
vtkSmartPointer<vtkPolyData>::New();
vtkSmartPointer<vtkPoints> boundsPoints =
vtkSmartPointer<vtkPoints>::New();
for( int i = 0 ; i < 8; ++i )
{
boundsPoints->InsertNextPoint( pts[i].point );
}
boundsPolydata->SetPoints( boundsPoints );
vtkSmartPointer<vtkCellArray> cells =
vtkSmartPointer<vtkCellArray>::New();
vtkIdType cell[2] = { 0, 1 };
cells->InsertNextCell( 2, cell );
cell[0] = 0; cell[1] = 2;
cells->InsertNextCell( 2, cell );
cell[0] = 3; cell[1] = 2;
cells->InsertNextCell( 2, cell );
cell[0] = 3; cell[1] = 1;
cells->InsertNextCell( 2, cell );
cell[0] = 4; cell[1] = 5;
cells->InsertNextCell( 2, cell );
cell[0] = 4; cell[1] = 6;
cells->InsertNextCell( 2, cell );
cell[0] = 7; cell[1] = 5;
cells->InsertNextCell( 2, cell );
cell[0] = 7; cell[1] = 6;
cells->InsertNextCell( 2, cell );
cell[0] = 1; cell[1] = 5;
cells->InsertNextCell( 2, cell );
cell[0] = 0; cell[1] = 4;
cells->InsertNextCell( 2, cell );
cell[0] = 2; cell[1] = 6;
cells->InsertNextCell( 2, cell );
cell[0] = 3; cell[1] = 7;
cells->InsertNextCell( 2, cell );
boundsPolydata->SetLines( cells );
return boundsPolydata;
}
Surfaces:
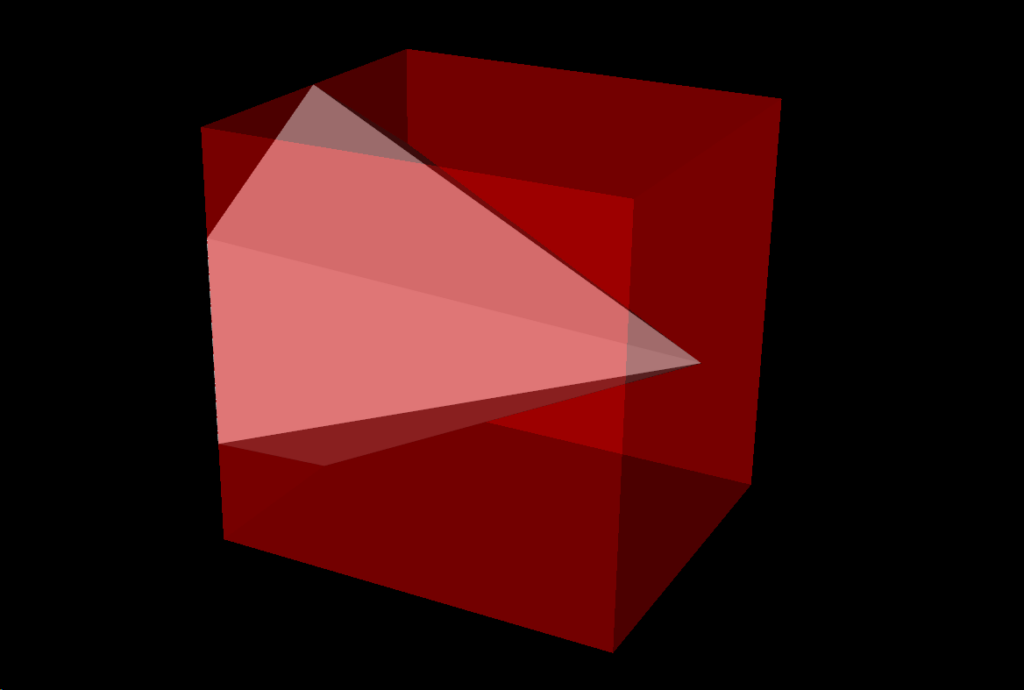
.
Lines:
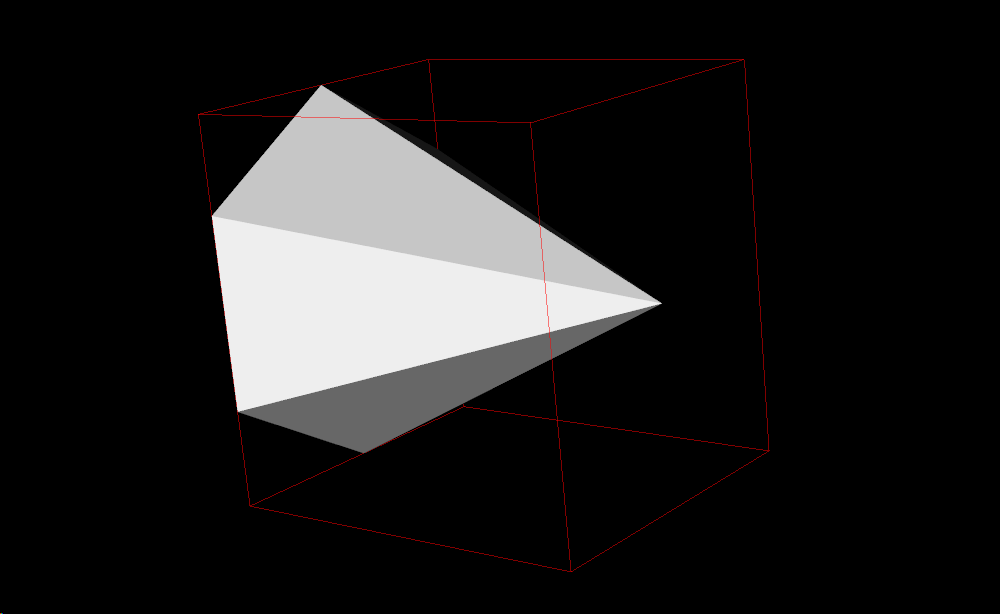
.
Complete code:
#include <iostream>
#include <vtkSmartPointer.h>
#include <vtkSphereSource.h>
#include <vtkActor.h>
#include <vtkConeSource.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkPolyDataMapper.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkParametricFunctionSource.h>
#include <vtkParametricTorus.h>
#include <vtkProperty.h>
#include "point.hpp"
#define vtkSPtr vtkSmartPointer
#define vtkSPtrNew(Var, Type) vtkSPtr<Type> Var = vtkSPtr<Type>::New();
using namespace std;
vtkSPtr<vtkPolyData> GetBoundsFacePolyData(double bounds[6])
{
double x[8][3]={{bounds[0],bounds[2],bounds[4]}, {bounds[1],bounds[2],bounds[4]}, {bounds[1],bounds[3],bounds[4]}, {bounds[0],bounds[3],bounds[4]},
{bounds[0],bounds[2],bounds[5]}, {bounds[1],bounds[2],bounds[5]}, {bounds[1],bounds[3],bounds[5]}, {bounds[0],bounds[3],bounds[5]}};
vtkIdType pts[6][4]={{0,1,2,3}, {4,5,6,7}, {0,1,5,4},
{1,2,6,5}, {2,3,7,6}, {3,0,4,7}};
vtkSPtrNew( points, vtkPoints );
vtkSPtrNew( polys, vtkCellArray );
vtkSPtrNew( polyData, vtkPolyData );
for (int i=0; i<8; i++) points->InsertPoint(i,x[i]);
for (int i=0; i<6; i++) polys->InsertNextCell(4,pts[i]);
polyData->SetPoints(points);
polyData->SetPolys(polys);
return polyData;
}
vtkSPtr<vtkPolyData> GetBoundsPolyData(double bounds[6])
{
std::vector<Point> pts;
for( int i = 0; i < 2; ++i )
{
for( int j = 2; j < 4; ++j )
{
for( int k = 4; k < 6; ++k )
{
pts.push_back( Point( bounds[i], bounds[j], bounds[k] ) );
}
}
}
vtkSmartPointer<vtkPolyData> boundsPolydata =
vtkSmartPointer<vtkPolyData>::New();
vtkSmartPointer<vtkPoints> boundsPoints =
vtkSmartPointer<vtkPoints>::New();
for( int i = 0 ; i < 8; ++i )
{
boundsPoints->InsertNextPoint( pts[i].point );
}
boundsPolydata->SetPoints( boundsPoints );
vtkSmartPointer<vtkCellArray> cells =
vtkSmartPointer<vtkCellArray>::New();
vtkIdType cell[2] = { 0, 1 };
cells->InsertNextCell( 2, cell );
cell[0] = 0; cell[1] = 2;
cells->InsertNextCell( 2, cell );
cell[0] = 3; cell[1] = 2;
cells->InsertNextCell( 2, cell );
cell[0] = 3; cell[1] = 1;
cells->InsertNextCell( 2, cell );
cell[0] = 4; cell[1] = 5;
cells->InsertNextCell( 2, cell );
cell[0] = 4; cell[1] = 6;
cells->InsertNextCell( 2, cell );
cell[0] = 7; cell[1] = 5;
cells->InsertNextCell( 2, cell );
cell[0] = 7; cell[1] = 6;
cells->InsertNextCell( 2, cell );
cell[0] = 1; cell[1] = 5;
cells->InsertNextCell( 2, cell );
cell[0] = 0; cell[1] = 4;
cells->InsertNextCell( 2, cell );
cell[0] = 2; cell[1] = 6;
cells->InsertNextCell( 2, cell );
cell[0] = 3; cell[1] = 7;
cells->InsertNextCell( 2, cell );
boundsPolydata->SetLines( cells );
return boundsPolydata;
}
int main()
{
vtkSPtrNew( source, vtkConeSource );
source->Update();
auto pd = source->GetOutput();
pd->ComputeBounds();
double bds[6];
pd->GetBounds( bds );
auto bdsPd = GetBoundsPolyData( bds );
vtkSPtrNew( mapper, vtkPolyDataMapper );
mapper->SetInputData( pd );
vtkSPtrNew( actor, vtkActor );
actor->SetMapper( mapper );
vtkSPtrNew( bdsMapper, vtkPolyDataMapper );
bdsMapper->SetInputData( bdsPd );
vtkSPtrNew( bdsActor, vtkActor );
bdsActor->SetMapper( bdsMapper );
bdsActor->GetProperty()->SetColor( 1, 0, 0 );
bdsActor->GetProperty()->SetOpacity( 0.5 );
vtkSPtrNew( renderer, vtkRenderer );
renderer->AddActor(actor);
renderer->AddActor(bdsActor);
renderer->SetBackground( 0, 0, 0 );
vtkSPtrNew( renderWindow, vtkRenderWindow );
renderWindow->AddRenderer( renderer );
vtkSPtrNew( renderWindowInteractor, vtkRenderWindowInteractor );
renderWindowInteractor->SetRenderWindow( renderWindow );
renderer->ResetCamera();
renderWindow->Render();
renderWindowInteractor->Start();
return 0;
}