Configure the SharedArrayBuffer response header in the http.server module of the Python standard library to enable the server to support a web multithreading environment. Let’s support SharedArrayBuffer on web browser.
SharedArrayBuffer is a JavaScript object that allows multiple WebWorker threads to share the same memory space. This means that different threads can access and modify the same data simultaneously without having to copy the data or worry about data synchronization issues. SharedArrayBuffer enables us to perform multithreading programming in a more efficient way, thereby improving performance.
Python script for setting up http server:
# Configure the SharedArrayBuffer response header in the http.server module of the Python standard library.
import os
from http.server import HTTPServer, SimpleHTTPRequestHandler
class CustomRequestHandler(SimpleHTTPRequestHandler):
def __init__(self, *args, **kwargs):
super().__init__(*args, directory=os.getcwd(), **kwargs)
def send_head(self):
path = self.translate_path(self.path)
# Check whether the file exists and get its MIME type
if not os.path.exists(path) or not os.path.isfile(path):
return None
ctype = self.guess_type(path)
try:
f = open(path, 'rb')
except OSError:
self.send_error(404, "File not found")
return None
self.send_response(200)
self.send_header("Content-type", ctype)
fs = os.fstat(f.fileno())
self.send_header("Content-Length", str(fs[6]))
self.send_header('Cross-Origin-Embedder-Policy', 'require-corp')
self.send_header('Cross-Origin-Opener-Policy', 'same-origin')
self.end_headers()
return f
def run_server(host='127.0.0.1', port=4001):
server_address = (host, port)
httpd = HTTPServer(server_address, CustomRequestHandler)
print(f'Starting server on {host}:{port}...')
httpd.serve_forever()
if __name__ == '__main__':
run_server()
Python server.py:
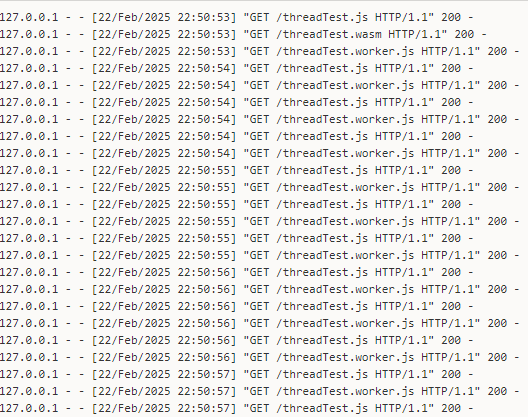